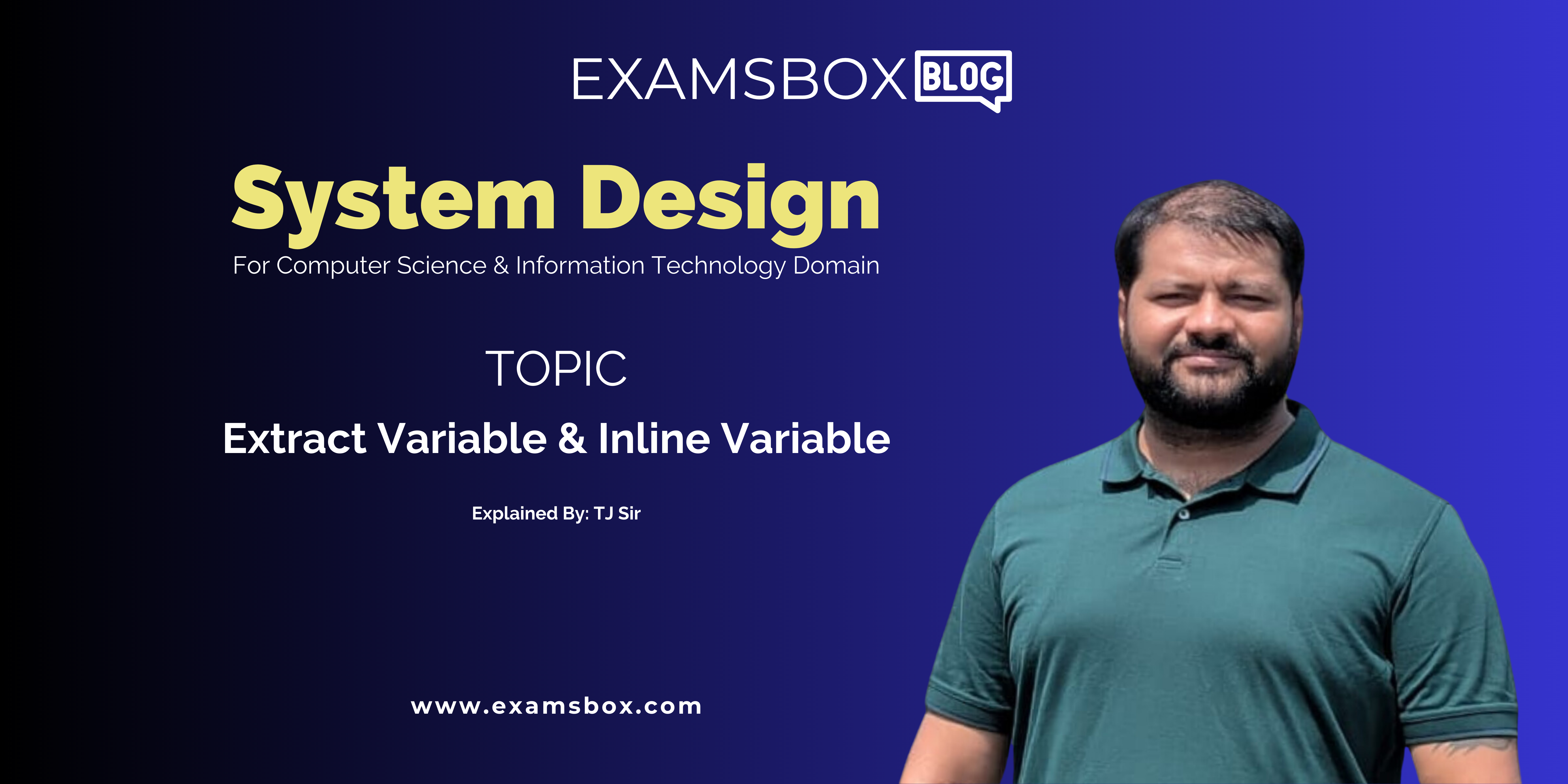
Extract Variable & Inline Variable
In software development, refactoring is an essential practice to enhance code quality. One common refactoring technique involves the use of variables. This blog post will guide you on when to use extract variable and inline variable techniques. You should extract a variable when a complex expression or piece of logic needs clarification. This technique helps improve readability and reusability. When a complicated expression could benefit from a more descriptive name. To avoid repetition and simplify maintenance. When a part of an expression is not immediately clear or could use clarification. Inline a variable when it doesn’t add much value and can simplify the code. When a variable holds a simple expression or constant that can be used directly. If the variable name doesn’t add meaningful context. When a variable is only used once, and its value is not complex.
Extract Variable helps break down complex logic into understandable parts with clear names.
Inline Variable reduces unnecessary variable declarations when the expression is simple and clear on its own.
// Before Extract Variable
if (user.age > 18 && user.hasLicense && !user.isSuspended) {
allowDriving();
}
// After Extract Variable
const canDrive = user.age > 18 && user.hasLicense && !user.isSuspended;
if (canDrive) {
allowDriving();
}
// Before Extract Variable
if (order.items.length > 0) {
processOrder(order.items.length);
}
log(order.items.length);
// After Extract Variable
const itemCount = order.items.length;
if (itemCount > 0) {
processOrder(itemCount);
}
log(itemCount);
// Before Extract Variable
if ((distance / time) > speedLimit) {
issueTicket();
}
// After Extract Variable
const speed = distance / time;
if (speed > speedLimit) {
issueTicket();
}
// Before Inline Variable
const discount = 0.1;
total = price * discount;
// After Inline Variable
total = price * 0.1;
// Before Inline Variable
const numberOfItems = cart.items.length;
if (numberOfItems > 0) {
checkout();
}
// After Inline Variable
if (cart.items.length > 0) {
checkout();
}
// Before Inline Variable
const isAdmin = user.role === 'admin';
if (isAdmin) {
showAdminPanel();
}
// After Inline Variable
if (user.role === 'admin') {
showAdminPanel();
}
Use Inline Variable when: